I'll go through step-by-step how to extract data from eBay listings using JavaScript. I'll explain each part of the code in detail.
Setup
We need to install two packages:
npm install request cheerio
And import them:
const request = require('request');
const cheerio = require('cheerio');
Define the eBay URL and headers:
const url = '<https://www.ebay.com/sch/i.html?_nkw=baseball>';
const headers = {
'User-Agent': 'Mozilla/5.0...' // Use your browser's UA string
};
Fetch the Listings Page
Use
request({url, headers}, (err, resp, html) => {
// Parse HTML here
});
Pass the url and headers, get the html in the callback.
Parse the HTML
Load html into Cheerio:
const $ = cheerio.load(html);
Cheerio parses HTML using jQuery style selectors.
Extract Data
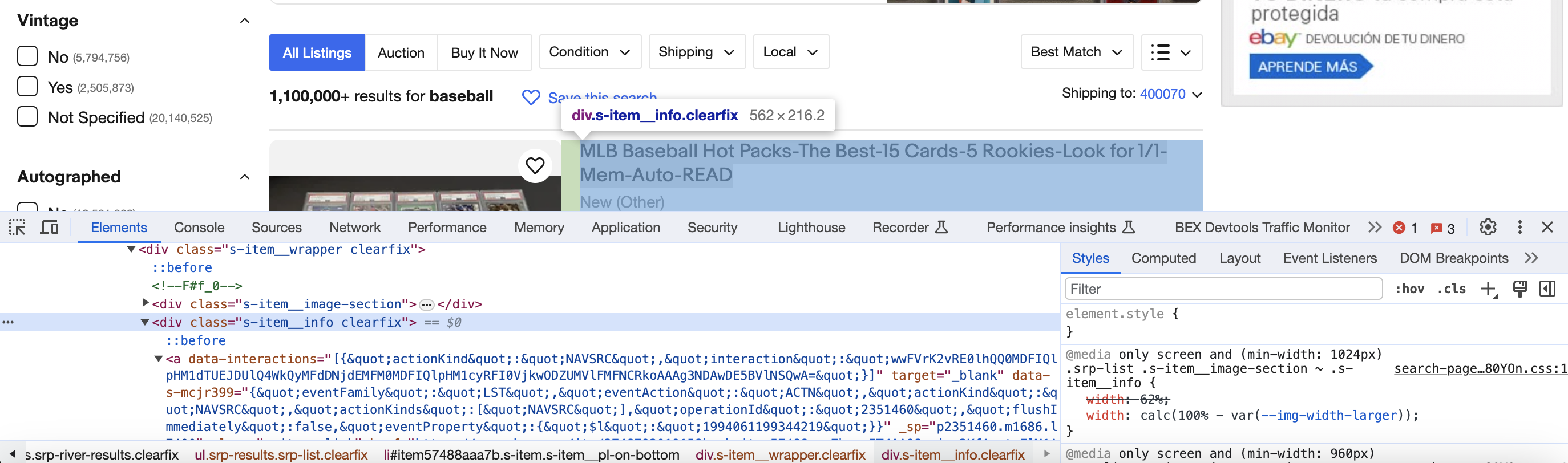
Loop through listing items:
$('.s-item__info').each((i, elem) => {
// Extract info from each listing item
});
Inside, extract text and attributes:
const title = $(elem).find('.s-item__title').text().trim();
const url = $(elem).find('.s-item__link').attr('href');
// etc for all fields
Print Results
console.log('Title: ' + title);
console.log('URL: ' + url);
console.log('='.repeat(50)); // Separator
Print each field.
Full Code
const request = require('request');
const cheerio = require('cheerio');
const url = 'https://www.ebay.com/sch/i.html?_nkw=baseball';
const headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.102 Safari/537.36'
};
request({url, headers}, (err, resp, html) => {
const $ = cheerio.load(html);
$('.s-item__info').each((i, elem) => {
const title = $(elem).find('.s-item__title').text().trim();
const url = $(elem).find('.s-item__link').attr('href');
const price = $(elem).find('.s-item__price').text().trim();
const details = $(elem).find('.s-item__subtitle').text().trim();
const seller = $(elem).find('.s-item__seller-info-text').text().trim();
const shipping = $(elem).find('.s-item__shipping').text().trim();
const location = $(elem).find('.s-item__location').text().trim();
const sold = $(elem).find('.s-item__quantity-sold').text().trim();
// Print all fields
console.log('Title: ' + title);
console.log('URL: ' + url);
console.log('Price: ' + price);
console.log('Details: ' + details);
console.log('Seller: ' + seller);
console.log('Shipping: ' + shipping);
console.log('Location: ' + location);
console.log('Sold: ' + sold);
console.log('='.repeat(50));
});
});