First, what exactly are "locators" in test automation? Simply put, locators allow us to uniquely identify elements on a web page so actions can be performed on those elements programmatically.
For example, imagine we have a login form with a username field, password field and login button. To automate logging in, we need a reliable way to target each element individually - locating them on the page.
Some common locator strategies include:
Each approach has pros and cons. ID locators work nicely when available - but dynamic apps rarely have stable IDs. Name locators also function well assuming devs implemented them properly. Class locators afford flexibility but can get convoluted.
So why should we care? Couldn't we just manually find elements and "hope for the best"? Well, no. Fragile element location leads to the automation disaster I experienced firsthand all those years back. Even minor application changes will destabilize test scripts relying on weak locators.
Maintaining hundreds of flaky UI checks is a test engineer's worst nightmare! It erodes confidence in automation and cripples the feedback cycle agile methodologies depend on.
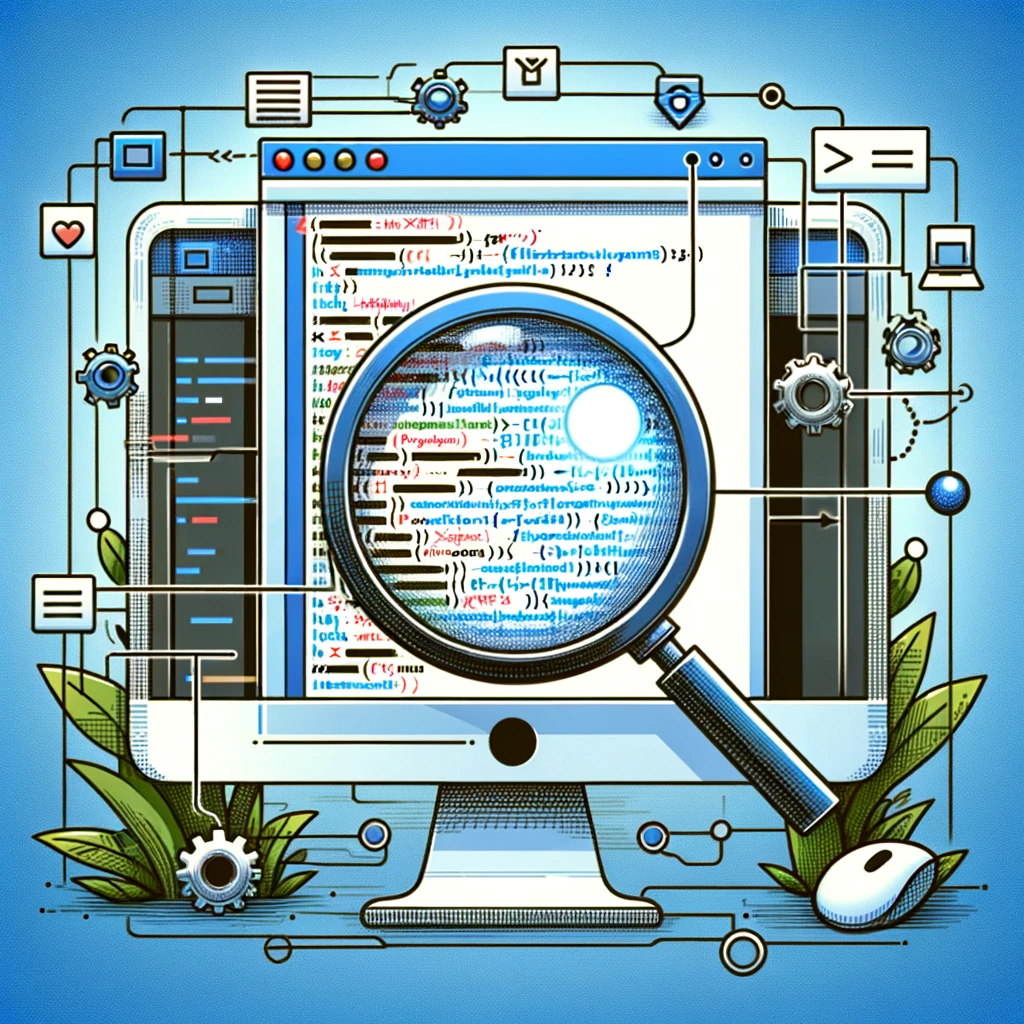
Mastering xpath locators - Types and Syntax
Clearly, robust reusable element location is critical for scaling test automation. For battle-hardened location, nothing beats XPath locators. Let's now demystify XPath to add this invaluable capability to our testing arsenal!
Absolute vs Relative XPath
The first key concept is the difference between absolute and relative XPath notation.
Absolute XPath refers to a full path from the root HTML element all the way down to the target node. For example:
/html/body/div/form/input
Relative XPath on the other hand allows us to start from any point within the nested structure. For example:
//form/input
Absolute XPaths are incredibly brittle. The slightest change at any level of the hierarchy breaks the locator. Relative XPaths affords much more flexibility. By anchoring to a nearby landmark then specifying the rest relationally, minor document tweaks are less likely to have catastrophic impacts.
Based on countless hours debugging broken scripts, my rule of thumb is to avoid absolute XPaths at all costs! Occasionally they are unavoidable, like when no other unique identifiers exist. But use relative notation whenever possible.
Advanced Syntax and Operators
Mastering basic XPath patterns is great - but the real power comes from employing advanced syntax and operators.
For example, we often need to match elements with partial text. The
//button[contains(text(),'Login')]
The above matches any button with "Login" text - ignore anything before or after.
Another common need is locating elements where we only know part of an attribute's value. Here's where the
//input[starts-with(@name,'user')]
This matches inputs whose name starts with "user" - so
Beyond text and attributes, we can also leverage logical operators like
//input[@type='text' and @name='q']
The above finds text input elements with name="q".
I could dive deeper but you get the point - with a strong grasp of XPath operators, we can construct dynamic locators to handle even the most complex test scenarios.
Integrating XPath Locators into Selenium Scripts
Now that we understand XPath fundamentals, let's shift gears and see how to integrate these locators into Selenium test automation scripts.
// Single element
WebElement elem = driver.findElement(By.xpath("//button[text()='Login']"));
// Multiple elements
List<WebElement> elems = driver.findElements(By.xpath("//input"));
The
Under the hood, Selenium bindings like Java leverage browser APIs to evaluate our XPath patterns against the current page's DOM structure. Results are returned for interaction in tests.
But there is a subtle yet critical difference between the singular vs plural methods - exception handling.
Singleton vs Multiple Elements
When locating a single element,
org.openqa.selenium.NoSuchElementException: no such element: Unable to locate element: {"method":"xpath","selector":""}
In contrast,
[]
Why does this matter? Because we must handle failures differently in our test logic.
For
try {
WebElement elem = driver.findElement(By.xpath("//h1"));
} catch (NoSuchElementException e) {
// Error handling logic
}
Whereas with
List<WebElement> elems = driver.findElements(By.xpath("//h1"));
if(elems.size() == 0) {
// Zero elements found
}
These subtle API differences can definitely trip you up. After hours spent debugging mysterious script failures, I've learned to pay close attention to exception handling with XPath locators.
Finding Multiple Elements
Another advantage of honing your XPath skills is the ability to fetch multiple elements in a single call. No need to separately locate each one.
Let's walk through a real-world example...
Say your application displays a grid of products, where each product is rendered as:
<div class="product">
<img src="product.jpg">
<span>Product Name</span>
<button>Add to Cart</button>
</div>
To add all products to the shopping cart, our script must:
- Identify each product
- Find Add to Cart button
- Click button
Rather than individually locating every product's button element, we can leverage a single XPath selector and
List<WebElement> addButtons = driver.findElements(By.xpath("//div[@class='product']/button")));
// Now iterate over list and click
for(WebElement btn : addButtons) {
btn.click();
}
This technique is extremely useful for repeating actions on dynamic collections of elements like data grids, product listings etc.
Best Practices for XPath Locator Reuse
Now that we have a firm grip on core XPath principles and usage in Selenium, I want to shift gears and cover some best practices I've learned over the years.
Most test engineers discover early on that XPath locators become extremely messy when scattered throughout scripts. Updates require touching tons of files - a maintenance nightmare!
The Page Object Model pattern helps alleviate this pain through locator reuse and encapsulation. Here's a simple example:
public class LoginPage {
private final By usernameLocator = By.xpath("//input[@id='username']");
private final By passwordLocator = By.xpath("//input[@id='pwd']");
private final By loginButtonLocator = By.xpath("//button[contains(text(),'Login')]");
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
}
public void setUserName(String user) {
driver.findElement(usernameLocator ).sendKeys(user);
}
public void setPassword(String pwd) {
driver.findElement(passwordLocator).sendKeys(pwd);
}
public void clickLoginButton() {
driver.findElement(loginButtonLocator).click();
}
}
Now tests simply interact with the
LoginPage login = new LoginPage(driver);
login.setUserName("test");
login.setPassword("abc123");
login.clickLoginButton();
If the devs ever change those elements, we only need to update locators in one spot!
While abstracting pages into classes takes more upfront effort, it pays back exponentially through easier test maintenance. Treat your locators as an investment rather than one-off code!
Diagnosing and Debugging XPath Issues
I'll wrap up this article by equipping you with troubleshooting skills for those inevitable XPath problems. Trust me - after hundreds of hours debugging flaky scripts - you WILL run into issues!
When a locator fails to find elements or your script starts throwing exceptions, how exactly do we debug? Here are 3 invaluable techniques:
1. Verify locator accuracy manually
Don't immediately assume your XPath expression is faulty. Oftentimes page state unexpectedly changes between steps.
Manually navigate to the target page and paste the selector into browser dev tools:
$x('//button[contains(text(),"Login")]')
If matching elements get highlighted, the query works fine - something else is going awry in the script flow.
2. Temporarily output attribute values
Another handy tactic is augmenting locators to dump out runtime attributes, especially for dynamic pages.
String txt = driver.findElement(By.xpath("//button[@type='submit']/@type")).getText();
System.out.println(txt);
Here we Grab the type attribute and print value. Does it match what you expect?
Attribute inspection reveals whether the located element truly is the intended target.
3. Try more resilient variations
Let's say the test platform URL changes subtly between test runs, breaking hardcoded locators.
Rather than fragile logic like:
//a[text()='<https://test.com/pricing>']
Make the pattern more robust:
//a[contains(text(), 'pricing')]
Now routing differences won't break our locator!
Moral of the story: Logic defensively and assume things will change. Building in flexibility takes a bit more work initially but prevents nightmare maintenance down the road
Key Takeaways
That wraps up my hard-earned lessons around mastering XPath locators for UI test automation using Selenium:
I hope walking through exactly how I leverage XPath locators day-to-day helps accelerate your automation efforts. Mastering these patterns is truly a milestone in transitioning from intermediate to expert-level test engineer.
The syntax and concepts can feel daunting initially. Stick with it through deliberate practice! It gets easier over time until locators become second-nature.
Frequently Asked Questions
Q: What's the fastest locator strategy in Selenium?
A: ID and name locators are typically fastest if implemented well in app code. XPath can sometimes get slow with complex expressions. But optimal speed depends on page structure - use browser profiling tools to identify worst performers.
Q: Is there a locators limit in Selenium?
A: No set locators limit exists. However, beware problems like stale element reference exceptions if trying to manage 1000s simultaneously. Bounding scope with pagination or search filtering is better than locating all elements upfront.
Q: Can I use CSS selectors instead of XPath in Selenium?
A: Absolutely! Selenium supports CSS just like XPath via By.cssSelector(). For simple cases CSS is great but XPath afford more flexibility for complex locators. Use each approach appropriately.