Web scraping can prove invaluable for gathering large volumes of data from websites. However, if done incorrectly, it also risks getting blocked by target sites. Using proxies with cURL in PHP provides an effective solution to circumvent blocks and scrape data successfully.
In this comprehensive guide, we will cover:
The Need for Proxies in Web Scraping
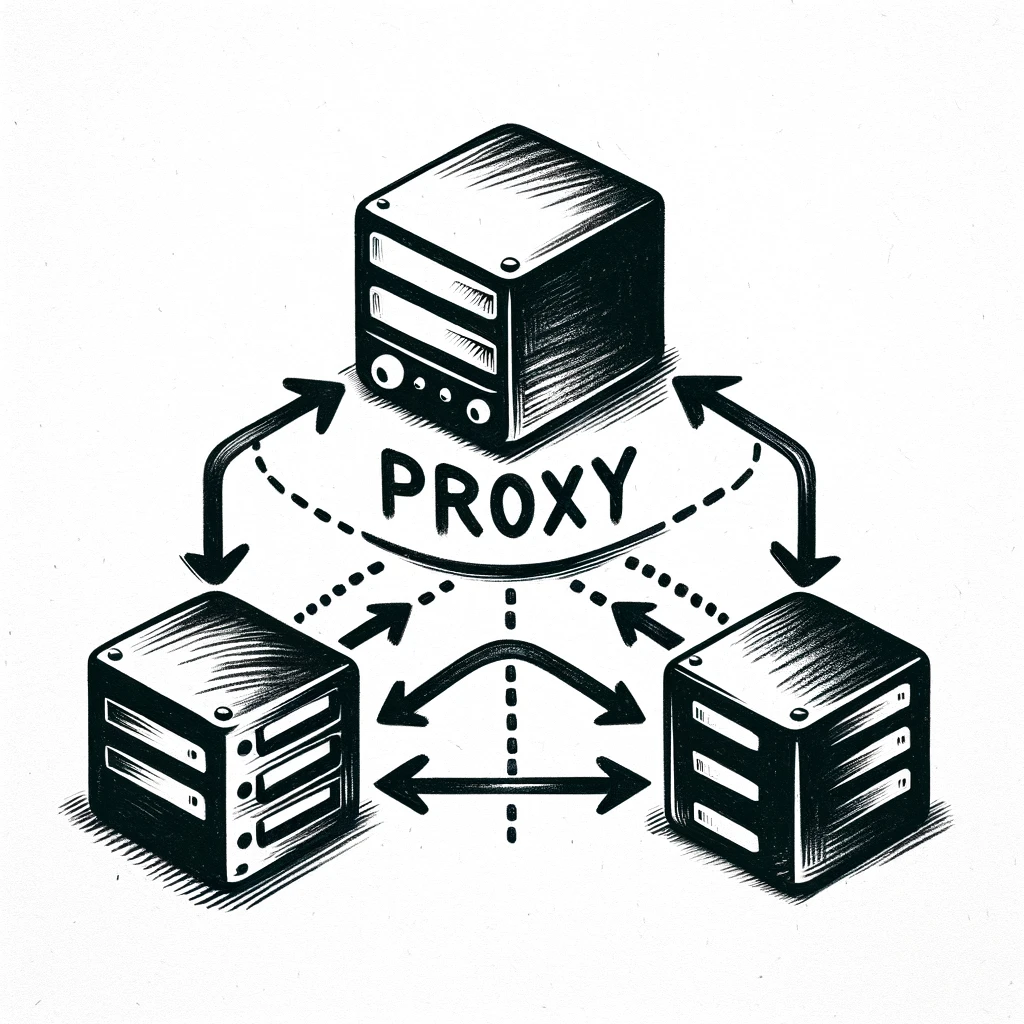
Now I'm sure many of you utilize web scraping for legitimate data collection purposes. However, most websites have anti-scraping mechanisms that trigger upon detecting unusual levels of activity from a single source.
So your perfectly written PHP scripts using cURL start getting blocked after a point, with fancy CAPTCHAs and firewall rules hampering your scraping initiatives.
Using proxies is an effective approach in this situation. Proxies basically mask your web scraper's identity by routing requests through intermediate servers. This makes your traffic seem more spread out and human-like, avoiding getting flagged as a scraper bot.
I faced plenty of blocks when scraping complex sites like Facebook and Instagram in my early days. Adding proxy rotation improved resilience and efficiency dramatically.
So in this guide, I'll share the techniques I learned over years of web scraping experiments with PHP cURL.
Section 1 - Basics of Using Proxies with PHP cURL
The first step is to understand the basic syntax for incorporating a proxy server with cURL in PHP.
Let's take an example:
$proxy = '123.45.6.7:8080';
$ch = curl_init('<http://www.example.com>');
curl_setopt($ch, CURLOPT_PROXY, $proxy);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$result = curl_exec($ch);
curl_close($ch);
Here
Some key points:
You can also set up proxy authentication in PHP cURL like so:
$proxy = '123.45.6.7:8080';
$proxyauth = 'username:password';
//...
curl_setopt($ch, CURLOPT_PROXY, $proxy);
curl_setopt($ch, CURLOPT_PROXYUSERPWD, $proxyauth);
The main benefit of using proxies is anonymity. Your requests don't directly reveal source server details with proxies in place.
However, there are additional advantages like being able to bypass regional blocks. For instance, scraping sites like BBC and Craigslist which restrict access from certain countries gets easier with international proxies routed through allowed regions.
Debugging network issues also gets more transparent since problems faced through one proxy would work perfectly on another indicating broader infrastructure bottlenecks.
Section 2 - Advanced Proxy Configurations
Now while setting up a basic proxy is quite straightforward, optimizing proxies further involves some more configurations.
Let's take a look at some advanced tactics.
Using Rotating Proxies
A common issue faced is proxies getting overused and hitting usage limits imposed by providers. This throttles speeds.
Here's a reliable approach I developed to leverage rotating proxies:
$proxies = ['123.45.6.7:8080', '98.76.54.3.21', '157.88.99.11:9000' ];
//...proxy rotation logic
$proxy = $proxies[array_rand($proxies)];
curl_setopt($ch, CURLOPT_PROXY, $proxy);
Essentially, the script picks a random proxy from the list before making each request. This distributes loads evenly across the proxy pool.
I suggest having at least 10-50 proxies in the pool to hedge against blocks. Maintaining this pool does take more upfront effort however.
Comparing Proxy Types
In my experience, residential proxies work best from an anonymity perspective. That's because they utilize IPs assigned to home Wi-Fi networks making your scraper traffic seem more human.
The downside is they aren't as blazing fast as datacenter proxies hosted on dedicated machines in offshore locations. Those easily deliver speeds above 1 Gbps but anonymity does take a hit occasionally.
Mobile proxies are slower however can emulate phone and tablet traffic perfectly when scraping sites with mobile-focused UIs.
So choose proxy types based on your specific scraping needs. Blend them also like using residential proxies just to extract CSRF tokens before shifting to faster datacenter ones for actual content extraction.
Section 3 - Best Practices for Proxy Integration
When incorporating proxies in PHP web scraping, following some basic best practices goes a long way in maintaining effectiveness.
Here are some top tips:
Use environment variables for proxy credentials
Hard-coding usernames and passwords hampers proxy rotation capabilities. Instead do:
export PROXY_USER=myusername
export PROXY_PASS=1234@pwd
//PHP script
$proxyauth = getenv('PROXY_USER').':'.getenv('PROXY_PASS');
This keeps actual credentials abstracted from application code.
Format output using HTML parsing libraries
While proxies return raw HTML, piping it through libraries like Goutte and PHPHtmlParser structurizes data effectively for analysis:
$proxy = '123.12.13.14:9090';
$ch = curl_init();
//...proxy setup
$html = str_get_html(curl_exec($ch));
foreach($html->find('h2') as $heading) {
echo $heading->plaintext . PHP_EOL;
}
Enable CAPTCHA solving mechanisms
Occasionally your proxies may encounter CAPTCHAs too which hamper scraper uptime despite rotating IPs. Integrating specialized anti-CAPTCHA services Tailor your scraping infrastructure keeping these tips in mind and you should be able to gather data efficiently without disruptions.
Section 4 - Handling Common Blocking Scenarios
Inevitably even proxy rotation configurations sometimes fail when scraping complex sites. Let's discuss some common blocking causes and potential solutions:
JavaScript Redirection Tracking
Many sites use JavaScript redirection to detect bots. So your IP rotates but device fingerprints remain constant opening up to blocks.
Solution: Use tools like Puppeteer that runs browsers natively solving this issue. But increased resource needs make it challenging to scale.
Third-party Content Embedded
Sites frequently embed maps, videos etc. served from external domains enforcing stricter regional access.
Solution: Scrape the site's main domain through proxies while allowing direct embedded content access from tool's server itself. This however slows down scraping due to extra network hops.
Aggressive Bot Protection Vendors
Websites buy expensive commercial solutions like Distil Networks meticulously tracking many parameters to identify bots. This breaks most DIY scrapers.
Solution: Outsource scraping to a managed service like Proxies API adept at evading advanced threats using in-house expertise and infrastructure.
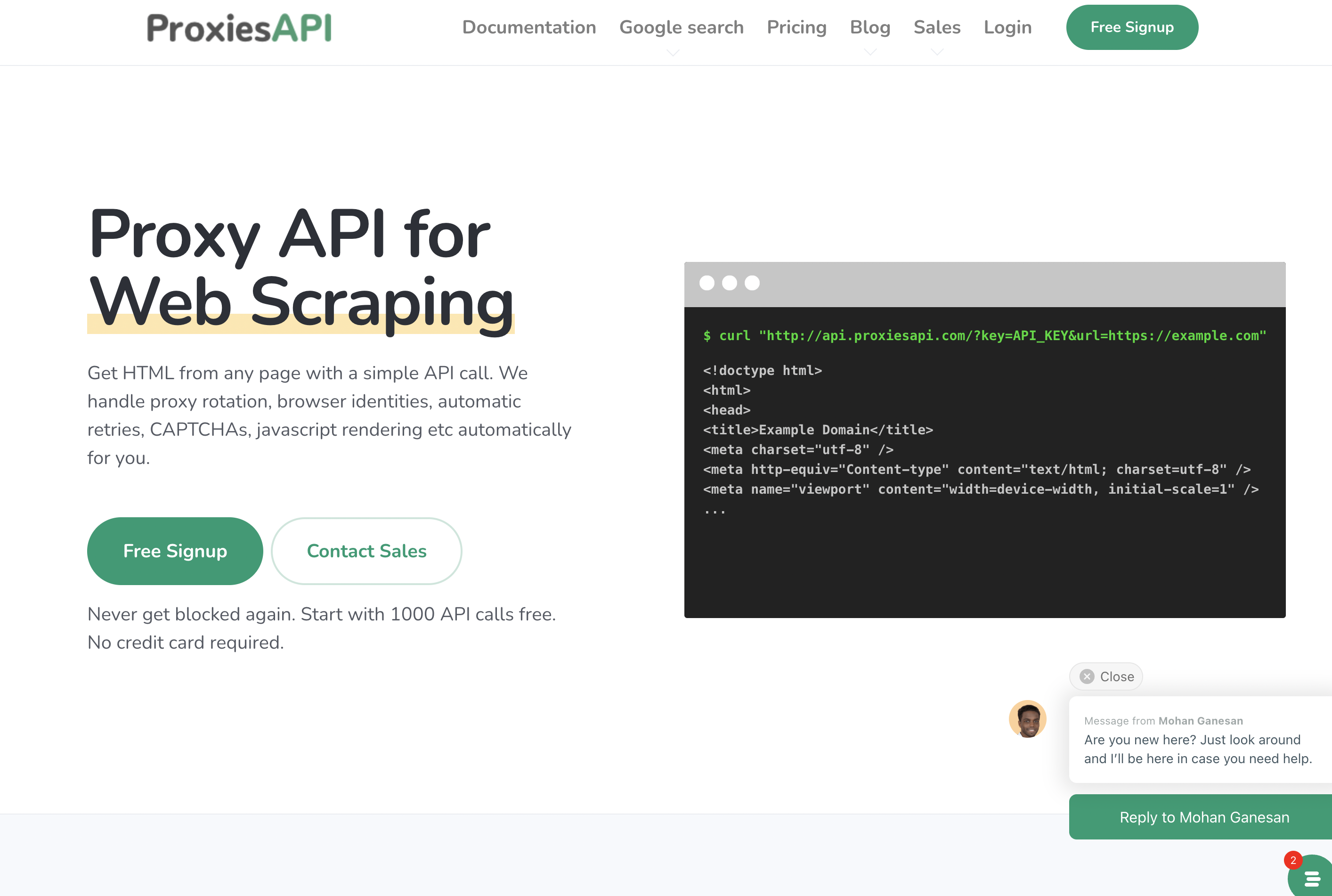
While the above examples highlight complex scenarios, our scraping proxy service Proxies API solves these issues behind the scenes without developers needing to bother about intricacies like rotation logic and CAPTCHA solving.
You get simple JSON/HTML back avoiding headaches of archaic blocks forcing you to focus on data analytics instead.
So I highly recommend checking out Proxies API for effortless, ad-hoc scraping with built-in anti-blocking capabilities. The first 1000 calls are free so it's worth exploring.